Uniapp 开发记录
Uniapp 开发记录
使用 Vue3 的 setup 语法糖
配置跨域代理
vue2
使用 HBuilderX 创建的项目
1 | // 文件 mainifest.json |
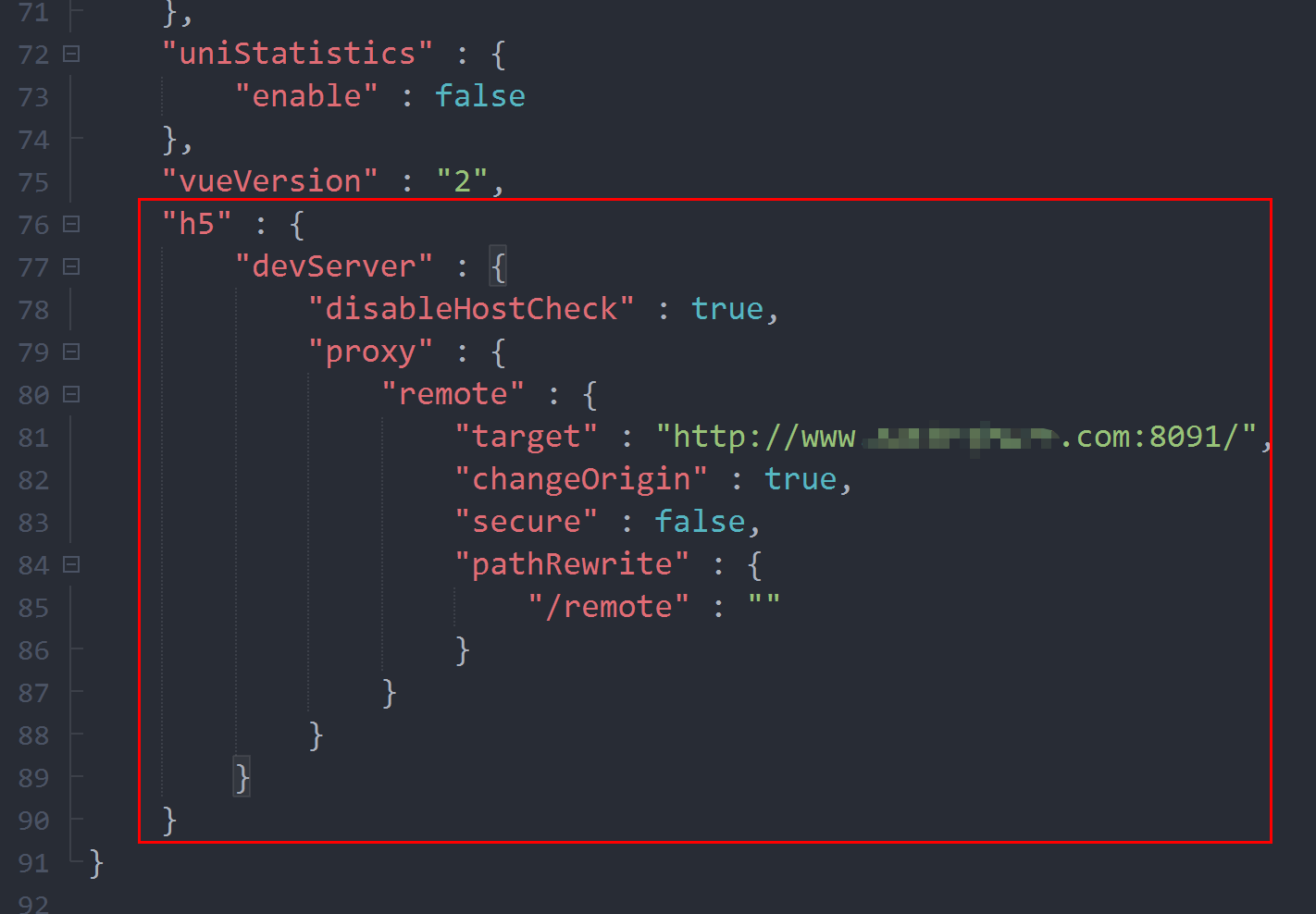
使用
1 | const url = '/remote/xxx/xxx' |
vue3 (无ts)
使用 HBuilderX 创建的项目
1 | // 在项目根目录创建 vite.config.js |
App 端无跨域问题,可以直接发送请求
应用生命周期
App.vue
是 uni-app 的主组件,所有页面都是在App.vue
下进行切换的,是页面入口文件。
但App.vue
本身不是页面,这里不能编写视图元素,也就是没有<template>
。
这个文件的作用包括:调用应用生命周期函数、配置全局样式、配置全局的存储globalData
应用生命周期仅可在App.vue
中监听,在页面监听无效。
1 | <script setup> |
条件编译
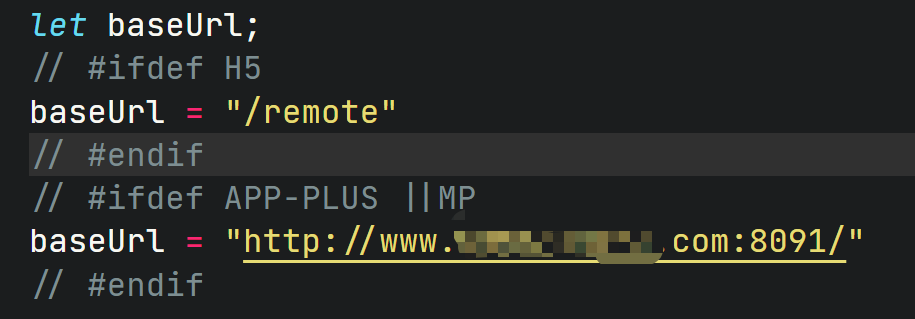
版本升级(整包更新)
函数 upgrade 需要放在 onLaunch 生命周期中
1 | import { useAppInfoStore } from '@/store/appInfo' |
相关 api :
[plus.runtime.getProperty()]: https://www.html5plus.org/doc/zh_cn/runtime.html#plus.runtime.version
1
2
3
4plus.runtime.getProperty(plus.runtime.appid, (widgetInfo) => {
console.log(widgetInfo.version)
})[uni.downloadFile()]: https://uniapp.dcloud.net.cn/api/request/network-file.html#downloadfile
1
2
3
4
5
6
7
8
9
10
11
12
13
14const downloadTask = uni.downloadFile({
url: xxx,
success: (downloadResult) = >{
console.log('下载成功, success', downloadResult)
},
fail: (err) = >{
console.log('downloadFile fail', err);
},
complete() { console.log('完成!') }
});
downloadTask.onProgressUpdate((res) = >{
console.log('下载进度' + res.progress);
});[plus.runtim.install(]: https://www.html5plus.org/doc/zh_cn/runtime.html#plus.runtime.install
1 | plus.runtime.install(downloadResult.tempFilePath, { |
配置底部导航 tabbar
效果:
.gif)
配置代码:
1 | { |
==pages 中需要有对应的页面==
App 端不支持 svg
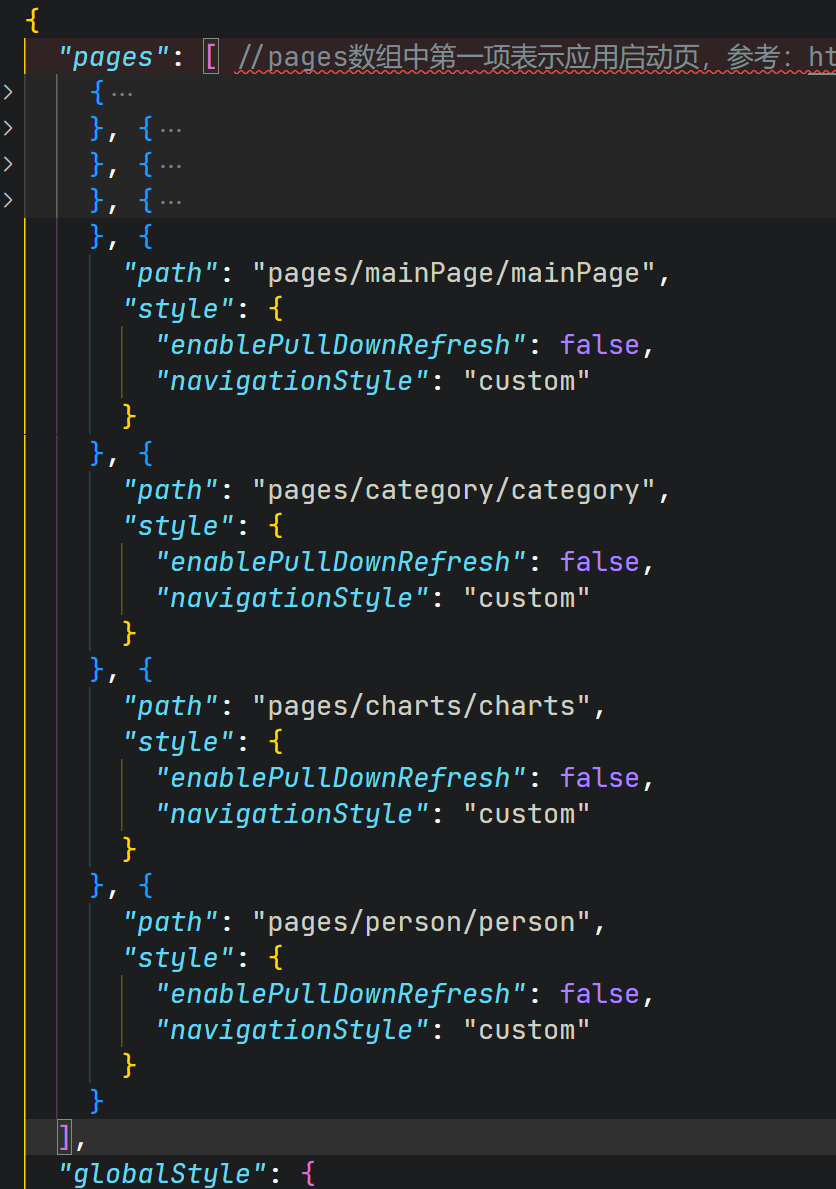
自定义顶部 top-bar
需要在 pages.json 中配置 "navigationStyle": "custom"
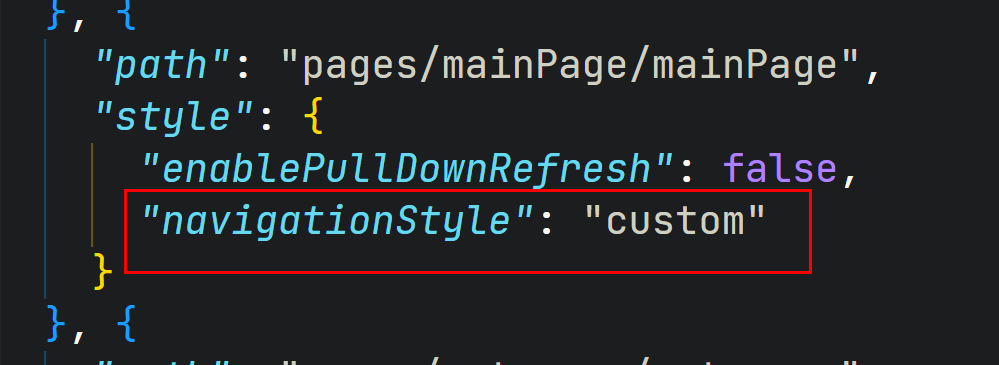
==手机端状态栏高度==
1 | <view class="status_bar"> <!-- 这是手机端最顶部的状态栏 --> </view> |